Google Apps Script Data Types, Variables, Special characters and Comments
Google Apps Script Data Types, Variables, Special characters and Comments
Numbers
Defining a number in Google Apps Script is actually pretty simple. The Number data type includes any
positive or negative integer, as well as decimals. Entering a number into the logger will return it right back to you.
function code1(){
var accountNumber=123456701;
Logger.log(accountNumber)
}
O/p=[18-02-15 15:21:05:813 IST] 1.23456701E8
Arithmetic operations
You can also perform calculations with numbers pretty easily. Basically type out an expression the way you would type it in a calculator.
function code2(){
var sum=1+3.2;
Logger.log(sum);
}
O/p=[18-02-15 15:21:06:813 IST] 4.2
Comparing numbers
Comparisons between numbers will either evaluate to true or false.
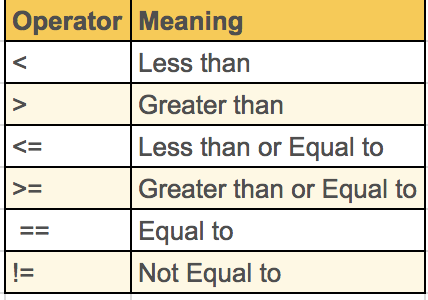
function code3(){
var comp=6>11;
Logger.log(comp);
}
o/p=[18-02-15 15:21:06:813 IST] false
function code4(){
var comp=6<11;
Logger.log(comp);
}
o/p=[18-02-15 15:39:59:907 IST] true
function code3(){
var comp=6>11;
Logger.log(comp);
}
o/p=[18-02-15 15:21:06:813 IST] false
function code4(){
var comp=6<11;
Logger.log(comp);
}
o/p=[18-02-15 15:39:59:907 IST] true
String
Strings are a collection of characters enclosed inside double or single quotes. You can use strings to represent data like sentences, names, addresses, and more.
function code6(){
// this is a single-line ‘comment’
var strNum=’1234567890’
Logger.log(strNum);
var strCon="Hello," + " World..."
Logger.log(strCon);
}
O/p [18-02-15 16:00:40:878 IST] 1234567890
[18-02-15 16:00:40:879 IST] Hello, World…
// this is a single-line ‘comment’
var strNum=’1234567890’
Logger.log(strNum);
var strCon="Hello," + " World..."
Logger.log(strCon);
}
O/p [18-02-15 16:00:40:878 IST] 1234567890
[18-02-15 16:00:40:879 IST] Hello, World…
Booleans
Very often, in programming, you will need a data type that can only have one of two values, like
YES / NO
ON / OFF
TRUE / FALSE
function Booleans(){
Logger.log(1<10)
}
O/p [18-03-11 18:31:44:175 IST] true
For this, Google Apps Script has a Boolean data type. It can only take the values true or false.
Null, Undefined, and NaN
Null: Value of nothing
Undefined: Absence of value in the given variable
NaN:NaN stands for "Not-A-Number"
function code8(){
var x=null;
var y;
var z="Shree"
Logger.log(" %s , %s , %s ",x,y,(z*10))
}
O/P: [18-02-15 17:45:33:927 IST] null , undefined , NaN
Special characters
If you want print "The file located at "C:\\Desktop\My Documents\Shree\data.txt" contains the names on the shree."
You need to write this
You need to write this
function code5(){
var splStr="The file located at \"C:\\\\Desktop\\My Documents\\Shree\\names.txt\" contains the names on the Shree.";
Logger.log(splStr)
}
O/p:[18-02-15 17:24:57:343 IST] The file located at "C:\\Desktop\My Documents\Shree\data.txt" contains the names on the shree.
"The file located at \"C:\\\\Desktop\\My Documents\\Shri\\data.txt\" contains the names on the shree."
Escape Characters list
Comments
You can use comments to help explain your code and make things clearer. In Google Apps Script, comments are marked with a double forward-slash //. Anything written on the same line after the // will not be executed or displayed. To have the comment span multiple lines, mark the start of your comment with a forward-slash and star, and then enclose your comment inside a star and forward-slash /* … */.
function code5(){
// this is a single-line comment
/* this is
a multi-line
Comment */
}
function code5(){
// this is a single-line comment
/* this is
a multi-line
Comment */
}
Happy Coding......
Comments
Post a Comment